You can call Python from C++ by embedding the Python interpreter and executing Python scripts or commands using the provided API.
Here's a simple code snippet demonstrating how to call a Python function from C++:
#include <Python.h>
int main()
{
Py_Initialize(); // Initialize the Python Interpreter
PyRun_SimpleString("print('Hello from Python!')"); // Execute a simple Python command
Py_Finalize(); // Finalize the Python Interpreter
return 0;
}
Understanding the Basics
C++ is a powerful, high-performance programming language favored for system-level programming, game development, and performance-critical applications. Python, on the other hand, is renowned for its simplicity and readability, making it a go-to language for scripting, machine learning, and data manipulation.
Why Call Python from C++?
The ability to call Python from C++ opens a realm of possibilities:
- Leverage Python Libraries: There are a plethora of established libraries in Python, especially in data science and machine learning, which can significantly speed up development.
- Integrate Different System Components: Utilization of C++ for performance-intensive tasks while leveraging Python’s scripting capabilities for rapid prototyping and higher-level logic.
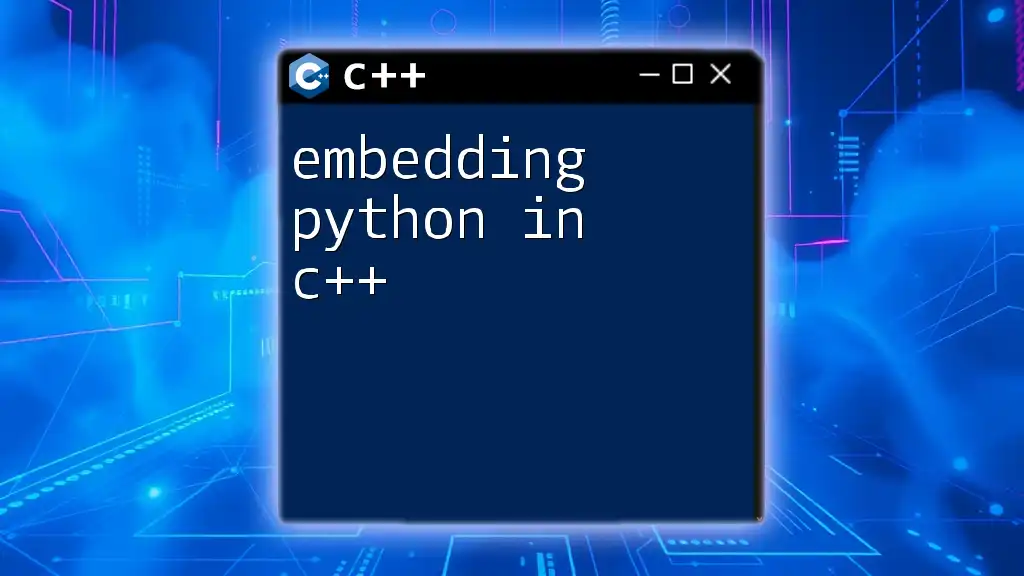
Setting Up Your Environment
Installing Python and C++
To get started, you need to have both C++ and Python installed on your system.
- Installing Python: Download Python from the official website and follow the installation instructions. Make sure to check the option to add Python to your PATH.
- Installing C++: Choose a suitable development environment, such as GCC, Clang, or Visual Studio, depending on your operating system.
Required Libraries and Tools
A few additional libraries can facilitate the interaction between C++ and Python:
-
Boost.Python: This library simplifies the interoperability and can be included in projects that already use Boost. Installation can be performed via package managers or compiling from source.
-
pybind11: A lightweight header-only library that offers seamless interoperability between C++ and Python. It can be installed using pip with the command:
pip install pybind11
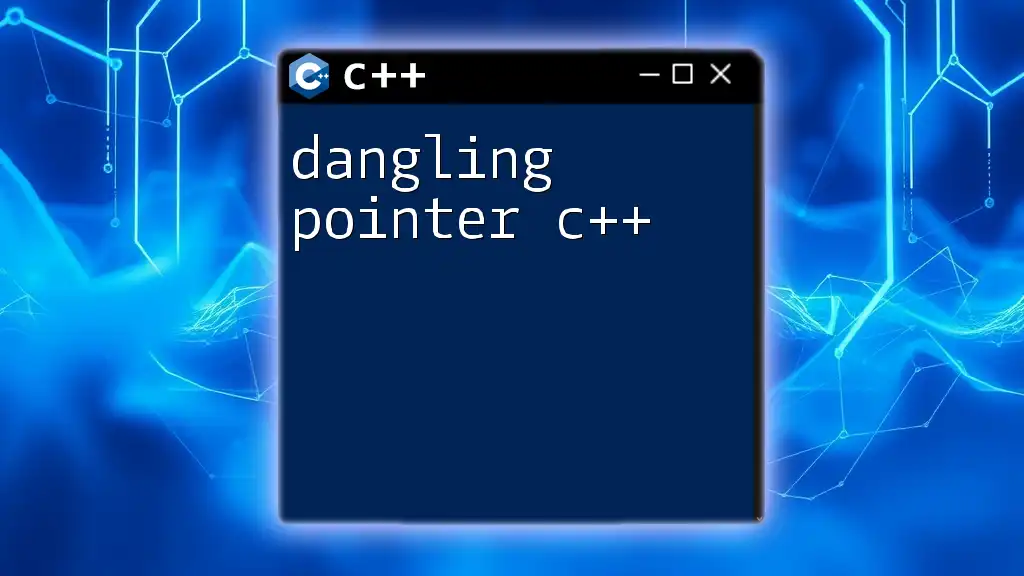
Methods to Call Python from C++
Using Python's C API
The Python C API allows direct interaction with Python code in C++. This method may seem complex but is powerful for lower-level control.
Example Code Snippet:
#include <Python.h>
int main() {
Py_Initialize();
PyRun_SimpleString("print('Hello from Python!')");
Py_Finalize();
return 0;
}
In this snippet, `Py_Initialize()` initializes the Python interpreter. The `PyRun_SimpleString()` function executes the given Python command, in this case, printing a message. Finally, `Py_Finalize()` cleans up and shuts down the interpreter.
Using Boost.Python
Boost.Python is a powerful library designed to give C++ programs the ability to call and manipulate Python code seamlessly.
Example Code Snippet:
#include <boost/python.hpp>
void greet() {
std::cout << "Hello from C++!" << std::endl;
}
int main() {
Py_Initialize();
boost::python::def("greet", greet);
Py_Finalize();
return 0;
}
In this case, we define a simple C++ function `greet()`, and using `boost::python::def()`, we expose it to Python. You can now call `greet()` from your Python code.
Using pybind11
pybind11 streamlines the process of calling Python from C++ with a focus on ease of use.
Example Code Snippet:
#include <pybind11/pybind11.h>
namespace py = pybind11;
void greet() {
std::cout << "Hello from C++!" << std::endl;
}
PYBIND11_MODULE(your_module_name, m) {
m.def("greet", &greet);
}
This module can be compiled and imported in Python. The `PYBIND11_MODULE` macro exposes the `greet()` function to the Python interpreter, allowing it to be called like any native Python function.
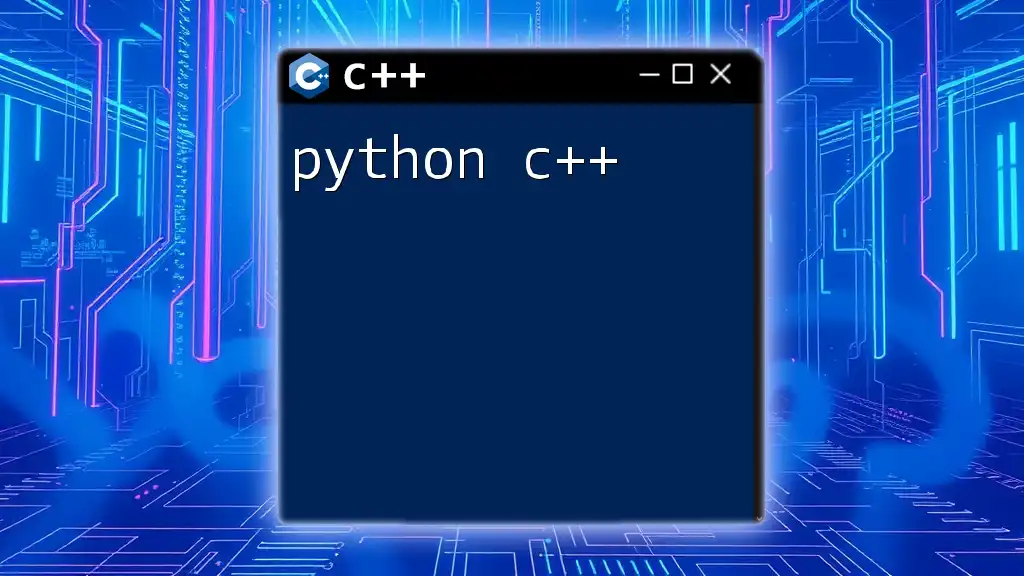
Practical Examples
Passing Data from C++ to Python
Data types are fundamental, and understanding how to convert them between C++ and Python is vital.
Example Code Snippet:
// Example for passing a list
#include <pybind11/pybind11.h>
namespace py = pybind11;
void pass_list() {
std::vector<int> values = {1, 2, 3, 4, 5};
py::list py_list;
for (int value : values) {
py_list.append(value);
}
// Now you can use py_list in your Python code
}
This code snippet demonstrates how to create a Python list from a C++ vector. The `append` method adds elements to the Python list.
Calling Python Functions from C++
When integrating systems, it is often necessary to call Python functions directly from your C++ code.
Example Code Snippet:
#include <boost/python.hpp>
int main() {
Py_Initialize();
try {
boost::python::object main_module = boost::python::import("__main__");
boost::python::object main_namespace = main_module.attr("__dict__");
// Assuming a Python script defines a square function
boost::python::exec("def square(x): return x * x", main_namespace);
boost::python::object result = main_namespace["square"](10);
std::cout << "Square: " << boost::python::extract<int>(result) << std::endl;
} catch (boost::python::error_already_set&) {
PyErr_Print();
}
Py_Finalize();
return 0;
}
This code snippet shows how to define a Python function within the main namespace and call it to calculate the square of a number.
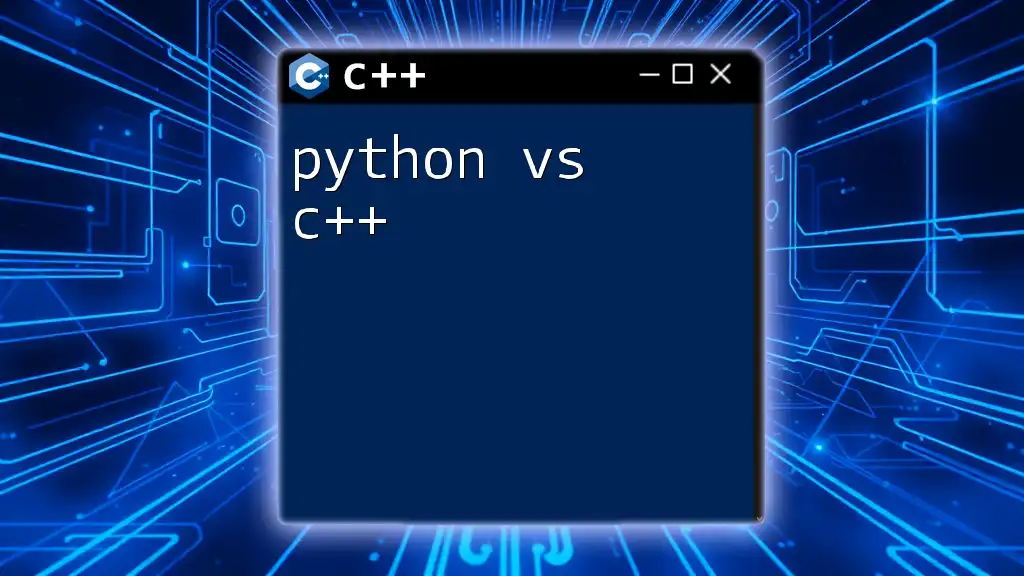
Error Handling
Common Pitfalls and Solutions
When calling Python from C++, you may run into various issues such as the Python interpreter not being initialized, or version mismatches between libraries.
Using Python Exception Handling in C++
It is crucial to catch exceptions originating from the Python code when called from C++ to maintain stability:
try {
// Call Python code
} catch (boost::python::error_already_set&) {
PyErr_Print(); // Print the error
}
This will allow you to debug by printing errors directly to the console.
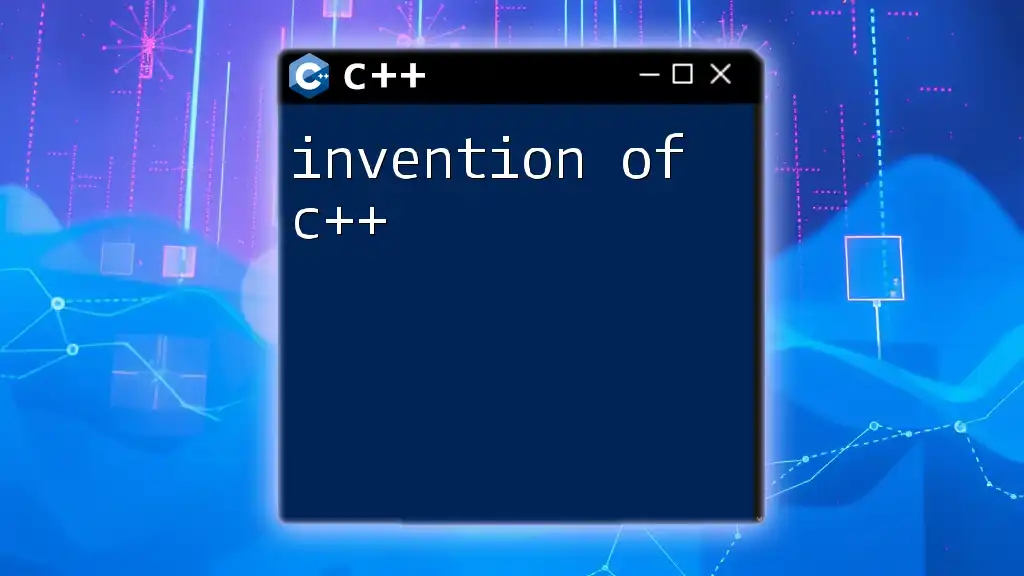
Best Practices
Optimizing Performance
To enhance communication speed between C++ and Python, consider limiting the number of calls between the two languages. Batch data transfers where possible.
Maintaining Code Readability
When integrating C++ and Python, clear separation between the logic in both languages improves maintainability. Use comments and proper naming conventions to keep your code understandable.
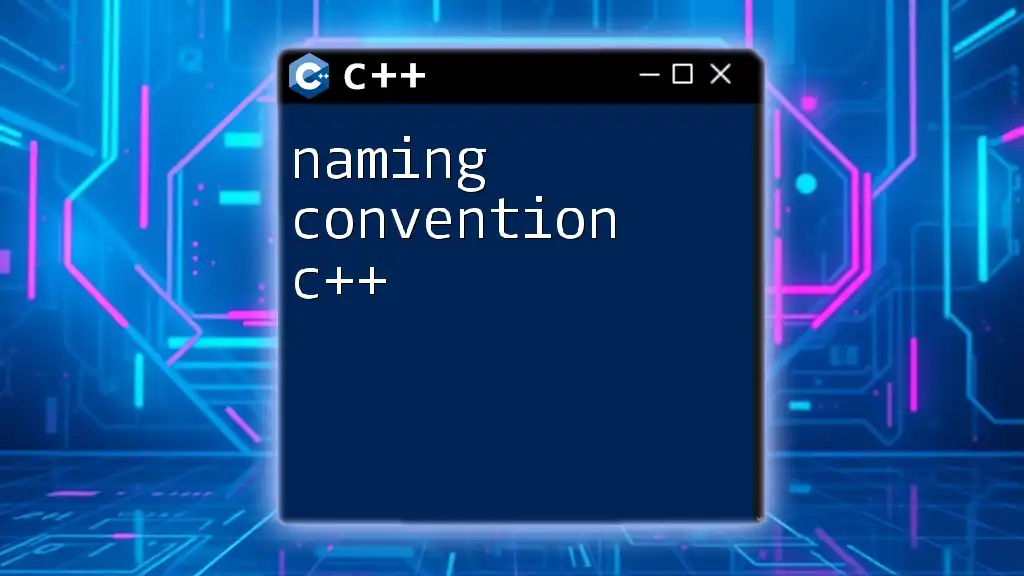
Conclusion
Calling Python from C++ is a powerful technique that allows developers to leverage the strengths of both languages. Whether you choose to use the Python C API, Boost.Python, or pybind11, each method has its unique benefits and workflows.
For further exploration, consider diving into the extensive documentation available for each library, and experiment with your own projects to fully grasp the concepts discussed.