C, C#, and C++ are distinct programming languages where C is a low-level procedural language, C# is a high-level object-oriented language designed for the .NET framework, and C++ is an extension of C that incorporates object-oriented features and supports both low-level and high-level programming.
Here's a simple code snippet that showcases similar functionality in each language:
// C example
#include <stdio.h>
int main() {
printf("Hello, World!\n");
return 0;
}
// C# example
using System;
class Program {
static void Main() {
Console.WriteLine("Hello, World!");
}
}
// C++ example
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C, C#, and C++
What is C?
C is a procedural programming language that was developed in the early 1970s at Bell Labs. As one of the oldest programming languages still in use, it has influenced many modern languages.
Key Features:
- Low-level access to memory, making it suitable for system programming.
- Portability and efficiency, leading to its widespread adoption in operating systems and embedded systems.
Common Use Cases: C is frequently used in contexts where direct control over hardware resources is necessary, such as:
- Operating systems (e.g., Linux)
- Embedded systems in consumer electronics
- Performance-critical applications
Code Example: Here's a simple C program that prints "Hello, World!" to the console:
#include <stdio.h>
int main() {
printf("Hello, World!");
return 0;
}
What is C#?
C# (pronounced "C sharp") is a modern programming language developed by Microsoft early in the 2000s as part of the .NET framework.
Key Features:
- Object-Oriented: C# embraces object-oriented programming, making it easier to manage large codebases.
- Rich Libraries: It provides a wide range of built-in libraries for handling various tasks, such as UI development and network communication.
Common Use Cases: C# excels in:
- Web development (especially with ASP.NET)
- Desktop applications for Windows
- Game development using Unity, popular for creating interactive experiences and simulations.
Code Example: Here’s a C# program that displays "Hello, World!" in the console:
using System;
class Program {
static void Main() {
Console.WriteLine("Hello, World!");
}
}
What is C++?
C++ is an extension of C that adds object-oriented features and was developed in the early 1980s.
Key Features:
- Object-Oriented Programming (OOP): C++ supports encapsulation, inheritance, and polymorphism, enabling developers to create complex and reusable code.
- Template Programming: C++ introduces templates for creating generic types, which promote code reusability and type safety.
Common Use Cases: C++ is used in various domains such as:
- Software development (e.g., Adobe products)
- Game engines (e.g., Unreal Engine)
- Scientific computing that requires high performance.
Code Example: This C++ program prints "Hello, World!" using the standard output:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}

Differences Between C, C++, and C#
Fundamental Differences
Programming Paradigms:
- C is primarily procedural, focusing on functions and a sequence of commands.
- C++ incorporates both procedural and object-oriented paradigms, allowing more complex data management.
- C# is entirely object-oriented, promoting modern programming practices and design patterns.
Memory Management:
- C requires manual allocation and deallocation of memory, which can lead to memory leaks if not handled correctly.
- C++ offers both manual management and constructors/destructors for cleanup.
- C# manages memory through garbage collection, automatically reclaiming unused memory, which can simplify development at the cost of performance overhead.
Syntax and Structure
Code Structure Differences: Each language represents information differently. C uses functions, while C++ introduces classes, and C# builds upon that with a more structured syntax that supports extensive library use.
Error Handling: C relies on return codes for error handling, requiring developers to check the outcome of function calls manually. In contrast:
- C++ provides exception handling to catch and handle errors more gracefully.
- C# features structured exception handling, simplifying error tracking and debugging.
Libraries and Frameworks
C relies on standard libraries, which provide essential functionality. C++, with its Standard Template Library (STL), offers powerful features for data structure management and algorithms.
C# shines with the extensive .NET library, providing built-in functionality for GUI creation, data access, web services, and more, heavily easing the development process in critical areas.
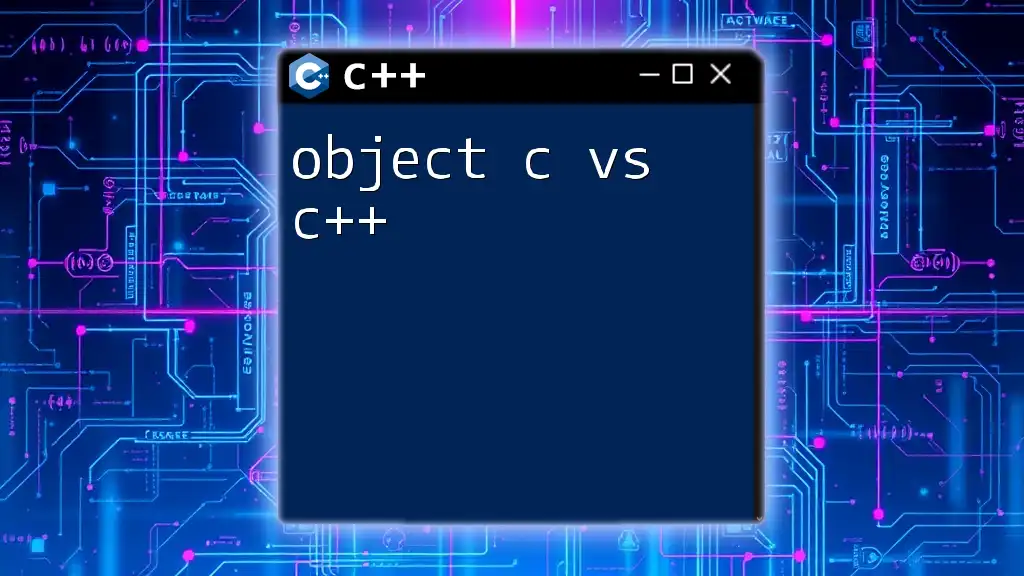
Performance and Efficiency
Memory Management and Performance
C's low-level memory management typically results in the highest performance, as programmers can optimize where necessary. C++ maintains similar performance but incurs slight overhead due to its object-oriented features.
C#, while simplifying development through garbage collection, can introduce performance overhead. Understanding these trade-offs is essential for performance-critical applications.
Compiler and Platform Dependency
C and C++ compile into machine code, lending themselves to performance optimizations. C# compiles into Intermediate Language (IL), which runs on the Common Language Runtime (CLR), providing cross-platform capabilities but slightly less performance efficiency in some instances.
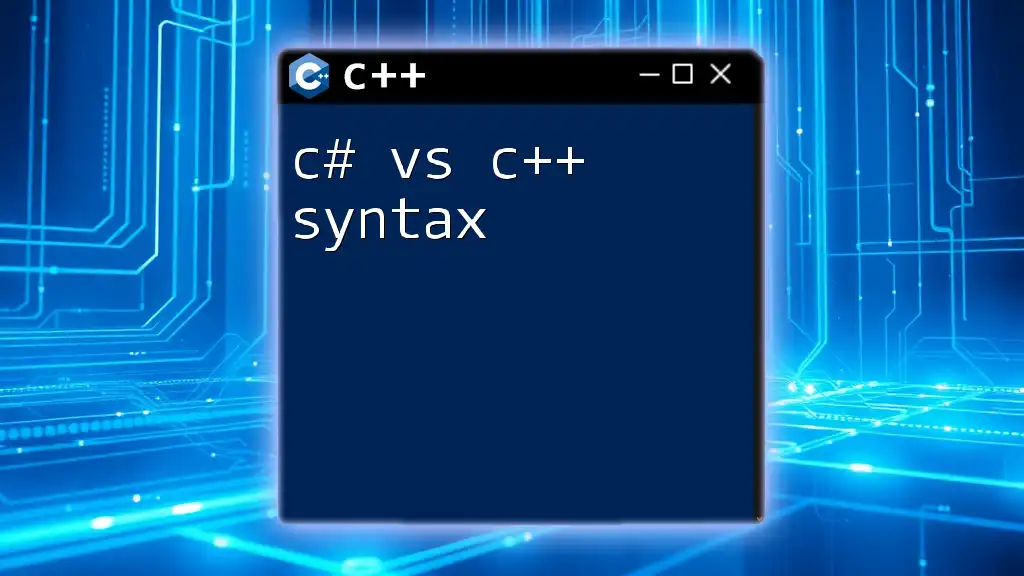
Community and Ecosystem
Popularity and Community Support
While C remains foundational, C++ has amassed a large community around it, primarily due to its use in game development and high-performance applications. C# has rapidly grown since its inception, especially within enterprise applications and game development (Unity).
Learning Resources
Many resources are available for learning each language:
- C has a wealth of classic textbooks and numerous online resources.
- C++ benefits from extensive forums and modern tutorials.
- C# enjoys Microsoft’s robust documentation and a large support community, significantly aiding learners.
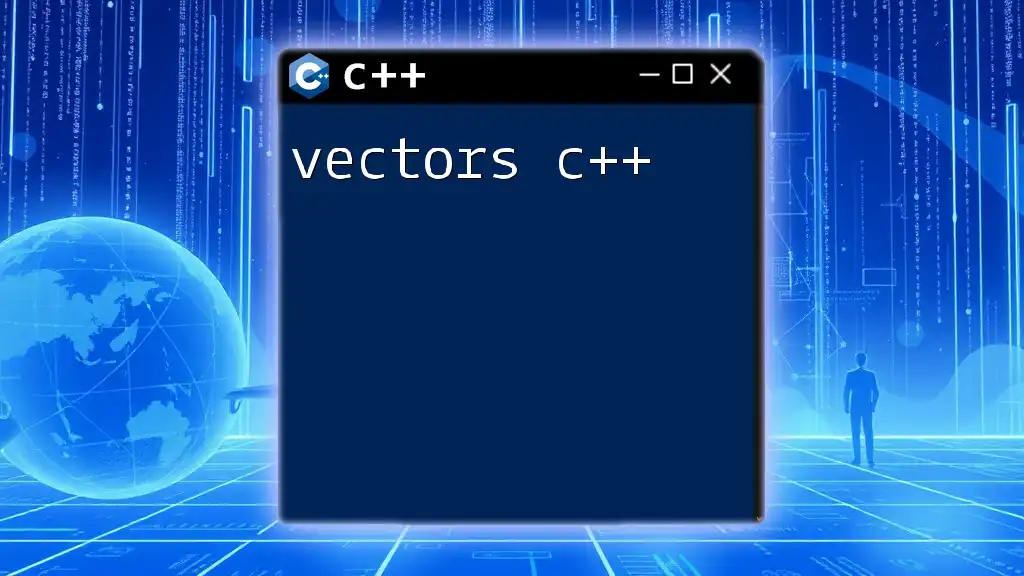
When to Use Which Language
Choosing the Right Language for Your Project
Selecting the right language depends on your project requirements:
- C is ideal if you need low-level hardware control or are developing resource-constrained systems.
- C++ is preferable for applications requiring performance optimization along with object-oriented design, such as graphical applications or large gaming projects.
- C# is well-suited for developing Windows applications, web services, or games using Unity, especially when rapid development is a priority.
In conclusion, understanding the distinctions among C, C++, and C# is critical for effectively choosing the right tool for your software development needs. Each language has its strengths and ideal use cases, so aligning your project goals with the right language can significantly enhance the quality and performance of the outcome.