Flowcharts and pseudocode are essential tools for mapping out the logic and structure of a C++ program before actual coding begins, enabling clearer understanding and efficient implementation.
Here's a simple code snippet demonstrating a basic C++ program structure that could be derived from flowchart and pseudocode planning:
#include <iostream>
using namespace std;
int main() {
int num1, num2, sum;
cout << "Enter two numbers: ";
cin >> num1 >> num2;
sum = num1 + num2;
cout << "Sum: " << sum << endl;
return 0;
}
What Are Flowcharts?
Definition of Flowcharts
Flowcharts are powerful visual tools that allow developers to understand and plan the structure of their programs before writing any code. They provide a clear graphical representation of a process, showcasing the flow of steps involved in solving a problem. By using specific shapes and symbols, flowcharts break down complex operations into understandable sections.
Components of Flowcharts
Flowcharts use various shapes to represent different types of actions or steps in a process:
- Oval: This shape indicates the Start and End points of the flowchart. It clearly shows where the process begins and terminates.
- Rectangle: Representing processes, rectangles hold the specific actions or calculations that need to occur.
- Diamond: This shape is used for decision points, guiding the flow based on yes/no questions or conditions.
- Parallelogram: Often used for Inputs and Outputs, this shape indicates where the program requires user input or where it displays results.
To illustrate these components, consider a simple flowchart for a calculator that adds two numbers. It begins with an oval for the Start point, followed by rectangles for inputting numbers and performing the addition. Finally, the end is marked with another oval.
Example Flowchart
Here's a basic representation of the flowchart logic for an addition calculator:
[Start] --> [Input num1, num2] --> [sum = num1 + num2] --> [Output sum] --> [End]
In C++, the corresponding structure might look like this:
// Simple C++ program to add two numbers
#include <iostream>
using namespace std;
int main() {
int a, b, sum;
cout << "Enter first number: ";
cin >> a;
cout << "Enter second number: ";
cin >> b;
sum = a + b;
cout << "Sum: " << sum << endl;
return 0;
}
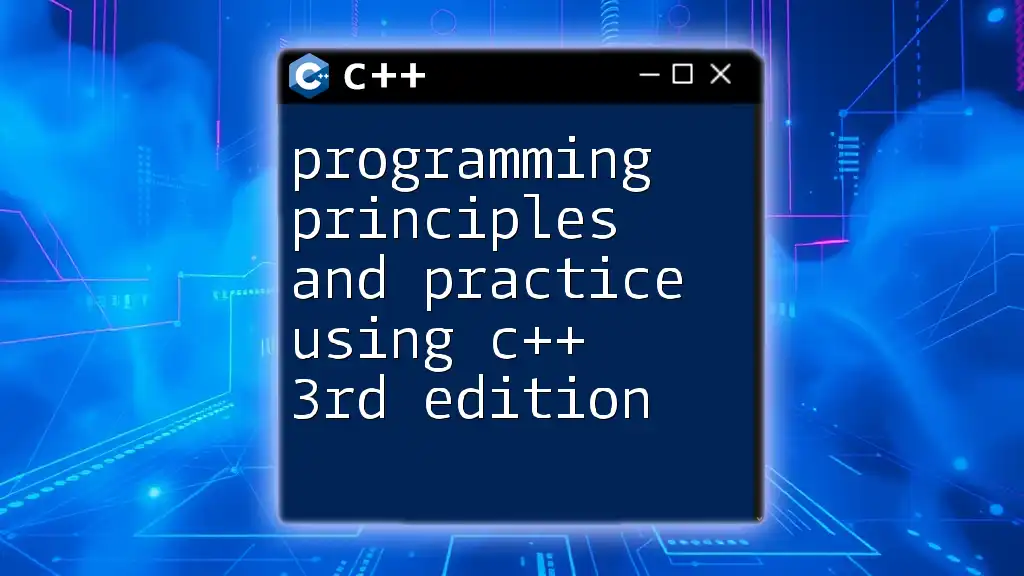
What Is Pseudocode?
Definition of Pseudocode
Pseudocode serves as a bridge between human reasoning and programming logic. It’s an informal way of representing a program’s logic using natural language statements and a simplified coding syntax. By employing pseudocode, programmers can focus on the algorithm’s flow without getting bogged down by the specific syntax of the programming language.
Key Features of Pseudocode
- Human-Friendly: Pseudocode is designed to be easily readable and understandable, making it accessible for individuals even without a programming background.
- Structured Format: Effective pseudocode maintains a clear structure through the use of indentation and keywords, which aligns closely with programming logic. Standard terms such as "IF", "FOR", and "WHILE" guide the structure.
Example of Pseudocode
For our addition calculation, the corresponding pseudocode can be expressed as follows:
START
Input num1, num2
sum = num1 + num2
Output sum
END
This pseudocode outlines the program's necessary steps in a concise manner, making it straightforward to translate into any programming language, including C++.
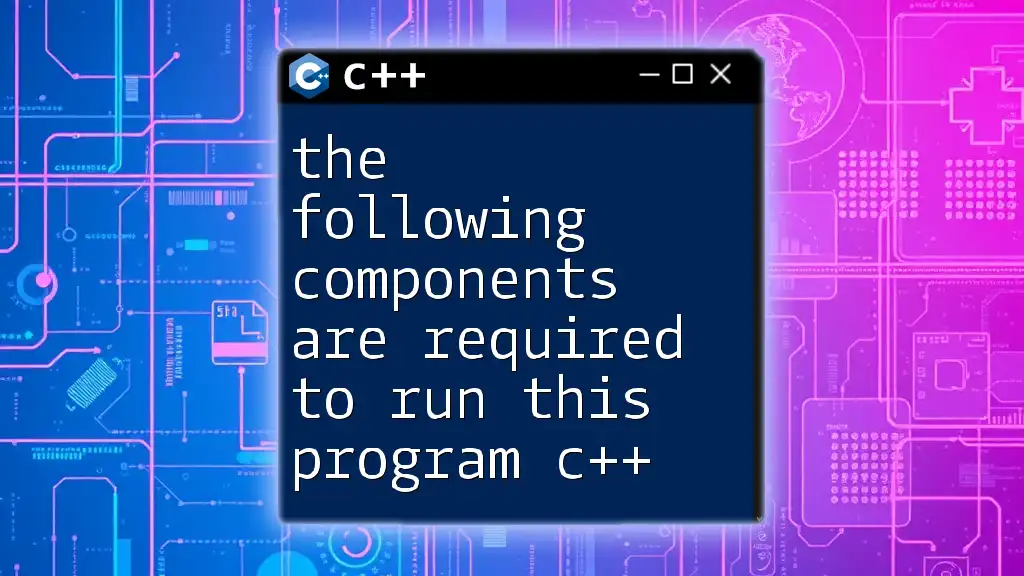
Benefits of Using Flowcharts and Pseudocode in C++
Simplifying Complex Problems
Using flowcharts and pseudocode allows developers to break down intricate problems into smaller, more manageable pieces. This is particularly useful when dealing with multi-step calculations or processes, such as developing a banking system that entails validating transactions, calculating interest, or maintaining account balances.
Enhancing Communication
These planning tools serve as excellent communication devices, particularly in team environments. Before diving into coding, flowcharts and pseudocode can help align team members, clarifying the project's direction for both technical and non-technical stakeholders. This ensures that everyone—designers, developers, and managers—understands the vision and logic of the program.
Error Reduction
Error management is another crucial advantage of using flowcharts and pseudocode. By mapping out the program logic before writing the code, developers can identify potential errors or logical flaws early in the process. This proactive approach reduces debugging time and simplifies subsequent coding phases.
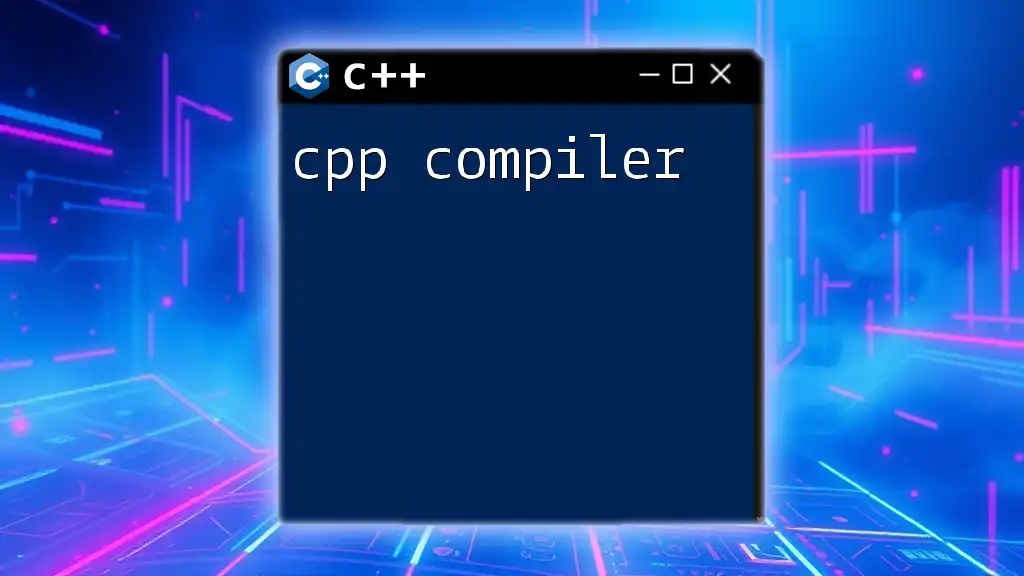
Steps to Create a C++ Program Using Flowcharts and Pseudocode
Step 1: Identify the Problem
Before any coding takes place, clearly understanding the problem at hand is vital. For example, when designing a loan approval system, it’s essential to determine the criteria for approval, user input requirements, and how outcomes should be communicated.
Step 2: Design the Flowchart
With the problem clearly defined, the next step is designing the flowchart. For the loan approval system, a flowchart might demonstrate how user inputs for income and loan amount guide the decision-making process. Decision points will delineate approval conditions, such as checking if the income meets a specific threshold.
Step 3: Write the Pseudocode
Next, draft the pseudocode to complement the flowchart. This step transforms visual logic into actionable text. The pseudocode for the loan approval might be as follows:
START
Input income, loanAmount
IF income > 50000 AND loanAmount < 200000 THEN
Output "Loan Approved"
ELSE
Output "Loan Denied"
END
Step 4: Translate to C++
Finally, translating the pseudocode to C++ involves coding it with the language’s syntax. For the loan approval example, the C++ program could be structured as follows:
// Loan Approval System in C++
#include <iostream>
using namespace std;
int main() {
double income, loanAmount;
cout << "Enter your income: ";
cin >> income;
cout << "Enter loan amount: ";
cin >> loanAmount;
if (income > 50000 && loanAmount < 200000) {
cout << "Loan Approved" << endl;
} else {
cout << "Loan Denied" << endl;
}
return 0;
}
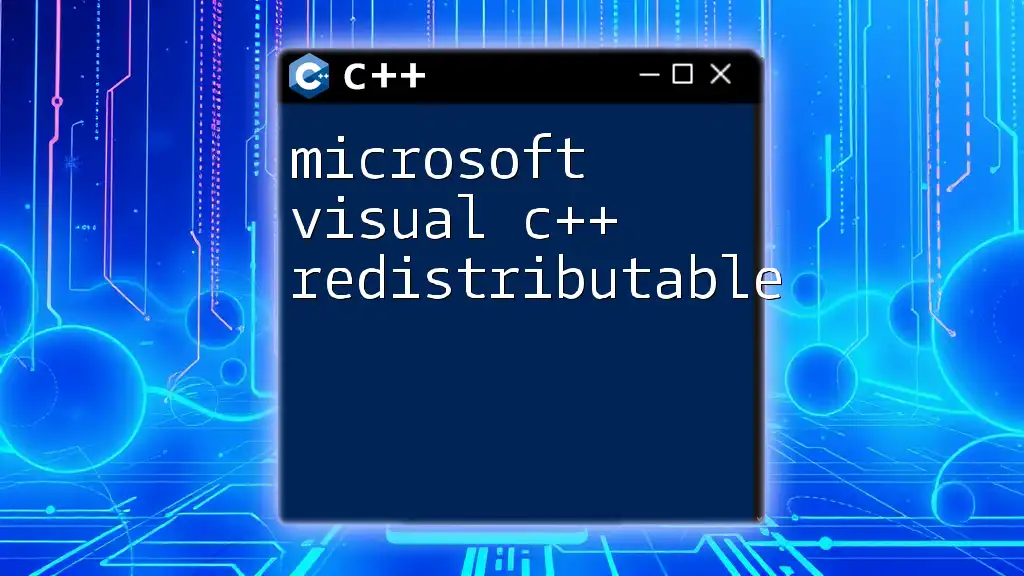
Best Practices for Using Flowcharts and Pseudocode
Keep It Simple
Keeping flowcharts and pseudocode simple enhances clarity and reduces misunderstandings. Avoid overcomplicating the graphical representations or using unnecessarily complex language. The goal is clear communication, so the final product should always focus on ease of understanding.
Consistency in Symbols and Terms
Establishing a consistent set of symbols and terminology across flowcharts and pseudocode is essential. This consistency prevents confusion, especially when multiple developers contribute to a project. It’s advisable to create a key for symbols and terms at the beginning of a project.
Review and Revise
Lastly, regular reviews of flowcharts and pseudocode ensure they remain relevant and accurate as the project evolves. As specifications change, updates should be made accordingly. Encouraging peer reviews further enhances the final product, providing an opportunity to catch overlooked errors and improve overall logic.
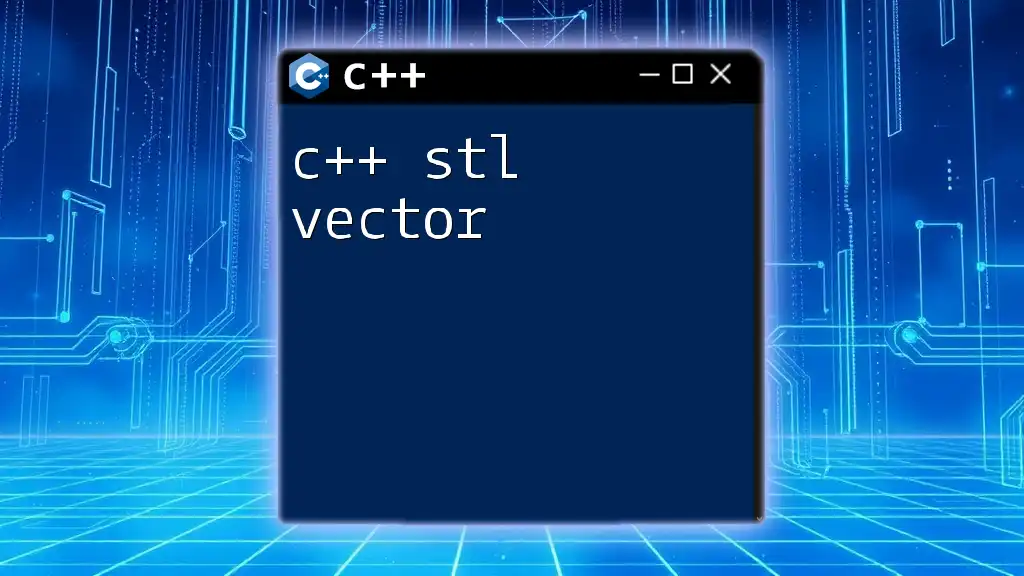
Tools for Creating Flowcharts and Pseudocode
Software Options
Numerous software tools can facilitate the creation of flowcharts and pseudocode. Some popular options include:
- Lucidchart: This online tool enables easy collaboration, making it simple for teams to work together in real time.
- Microsoft Visio: A more traditional option that offers extensive diagramming capabilities for both flowcharts and technical diagrams.
- Draw.io: A free, web-based platform that allows users to create flowcharts and various diagrams effortlessly.
Using Online Resources
Additionally, a wealth of online resources offers templates and guides for crafting flowcharts and pseudocode. Exploring these resources can provide helpful inspiration and accelerate the process of program planning.
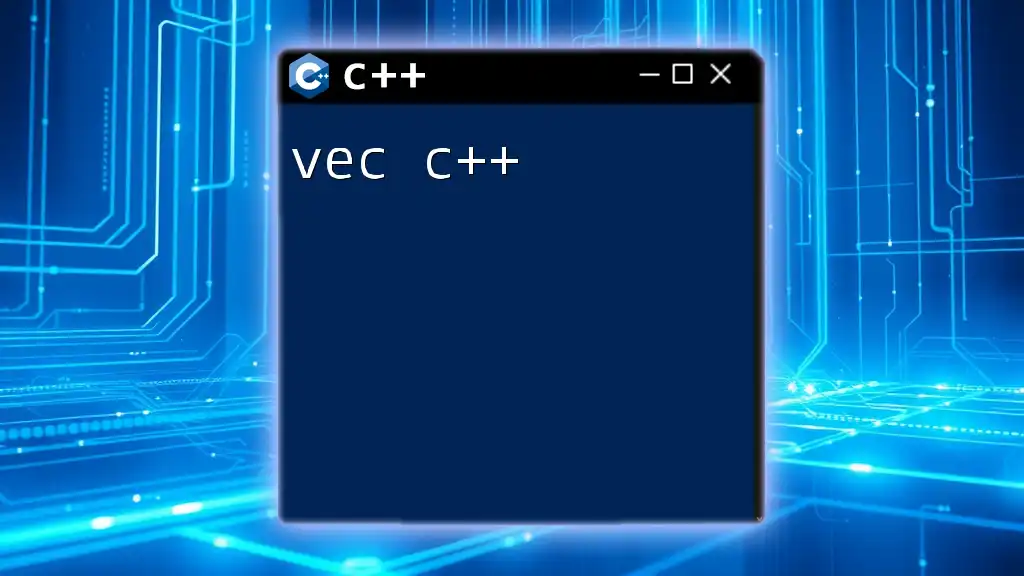
Conclusion
In conclusion, using flowcharts and pseudocode to write a C++ program not only simplifies the development process but also enhances communication, reduces errors, and fosters collaboration among team members. By taking the time to plan out logic through these tools, developers can effectively transform ideas into functional programs.
I encourage you to practice creating flowcharts and writing pseudocode before diving into coding. Start with simple projects, and as you gain confidence, tackle more complex systems. Remember, a well-planned program leads to clean, maintainable code. Happy programming!
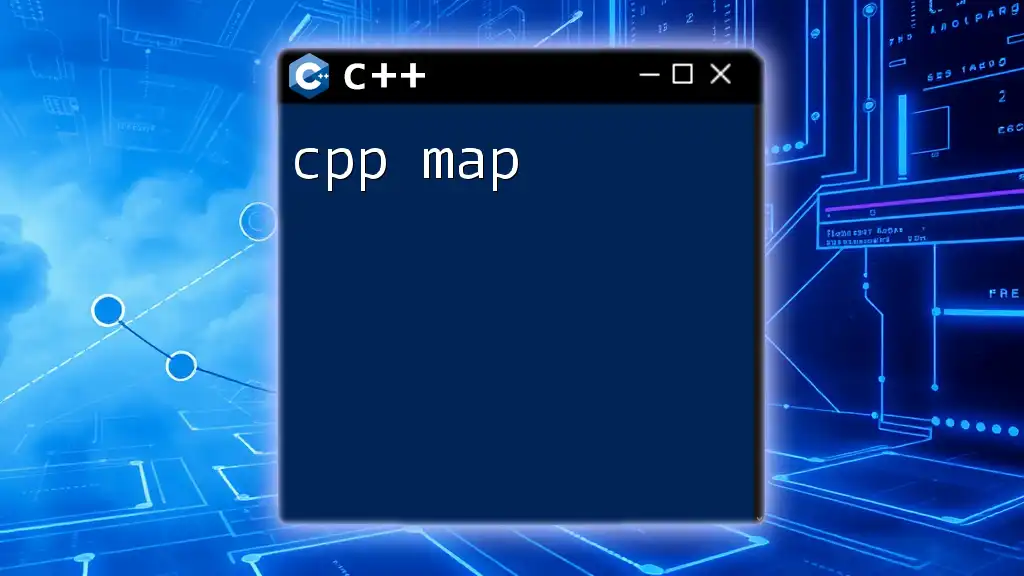
Additional Resources
For further reading, consider exploring online C++ documentation, and check out recommended books on flowcharting and pseudocode techniques to deepen your understanding and sharpen your skills.