To calculate the logarithm base 2 of an integer in C++, you can use the `log2` function from the `<cmath>` library, which returns the logarithm of a given number in base 2.
#include <iostream>
#include <cmath>
int main() {
int number = 16;
double logBase2 = log2(number);
std::cout << "Log base 2 of " << number << " is: " << logBase2 << std::endl;
return 0;
}
Understanding Logarithms
What is a Logarithm?
A logarithm is a mathematical function that helps us understand how many times one number must be multiplied by itself to obtain another number. In simple terms, the logarithm answers the question: "To what power do we raise a certain base to get a number?"
For example, in logarithmic terms, if you have \( 2^x = 8 \), you can express this as \( x = \log_2(8) \). This means 2 raised to the power of 3 gets you 8, so \( \log_2(8) \) equals 3.
Log Base 2 Explained
Logarithm base 2, denoted as \( \log_2(x) \), specifically measures how many times the number 2 must be multiplied together to achieve the number x. This function is particularly important in computing, as many algorithms and data structures rely on binary logic — using base 2 gives significant insight into their efficiency.
For instance, in computer science, algorithms that utilize binary searching, sorting, and data representation often involve the concept of logarithm base 2. Knowing how to compute the log base 2 of an integer helps in understanding the complexity of algorithms.
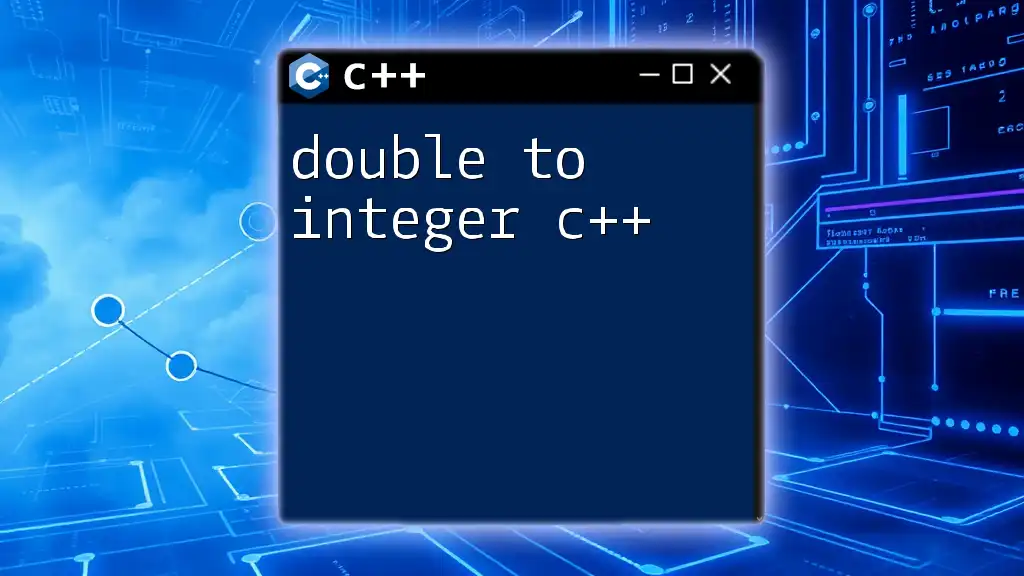
Calculating Log Base 2 in C++
Using Functions from the C++ Standard Library
Overview of `<cmath>`
The `<cmath>` library in C++ provides powerful mathematical functions that simplify performing complex calculations. Among these functions is `log2()`, specifically designed to compute the logarithm base 2 for a given number.
The `log2` Function
The syntax of the `log2` function is straightforward:
double log2(double x);
This function takes a double precision floating-point number as input and returns the log base 2 of that number. It's essential to ensure that x is greater than zero, as logarithm of zero or negative numbers is undefined.
Here’s a basic usage example:
#include <iostream>
#include <cmath>
int main() {
double number = 8.0;
double result = log2(number);
std::cout << "Log base 2 of " << number << " is: " << result << std::endl;
return 0;
}
Explanation: In this example, we include the `<cmath>` library for mathematical functions. We define `number` as 8.0 and then compute its log base 2 using the `log2` function. The result is printed, displaying "Log base 2 of 8 is: 3".
Implementing Your Own Log Base 2 Function
Why Implement Your Own?
While using `log2` from the `<cmath>` library is convenient and efficient, implementing your own log base 2 function offers a deeper understanding of the underlying math, as well as potential customization for specific needs in your projects.
Using Bit Manipulation
Bit manipulation provides a unique approach to calculate the log base 2. By leveraging the binary representation of integers, you can efficiently determine how many times you can divide a number by 2 until you reach 1.
Here’s an example of a custom implementation:
#include <iostream>
int customLog2(int n) {
int log = 0;
while (n >>= 1) {
log++;
}
return log;
}
int main() {
int number = 16;
std::cout << "Log base 2 of " << number << " is: " << customLog2(number) << std::endl;
return 0;
}
Explanation: In this code, the function `customLog2` shifts the bits of `n` to the right (essentially dividing by 2) until `n` becomes zero, incrementing the `log` counter each time. When we call `customLog2(16)`, it returns 4, illustrating that \( 2^4 = 16 \).
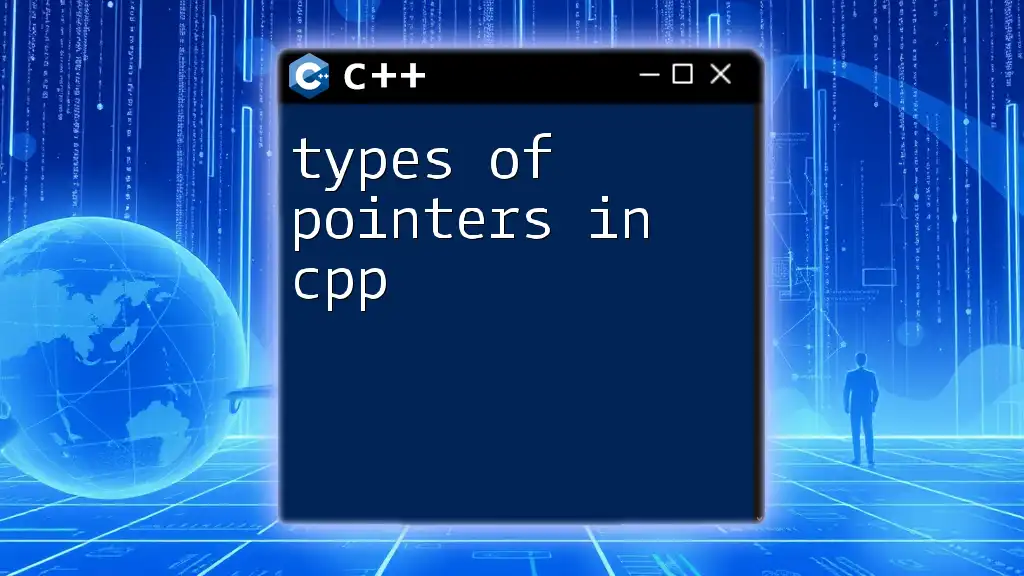
Handling Edge Cases
Logarithm of Zero and Negative Numbers
Mathematically, the logarithm of zero or any negative number is undefined. The `log2` function in C++ will return a domain error in such cases, resulting in `NaN` (Not a Number) when calculating:
Here’s how to handle this edge case in C++:
#include <iostream>
#include <cmath>
int main() {
double number = 0.0;
if (number <= 0) {
std::cout << "Logarithm undefined for non-positive numbers!" << std::endl;
} else {
std::cout << "Log base 2 of " << number << " is: " << log2(number) << std::endl;
}
return 0;
}
In this snippet, we check if `number` is less than or equal to zero before attempting to calculate the logarithm, thereby avoiding undefined behavior.
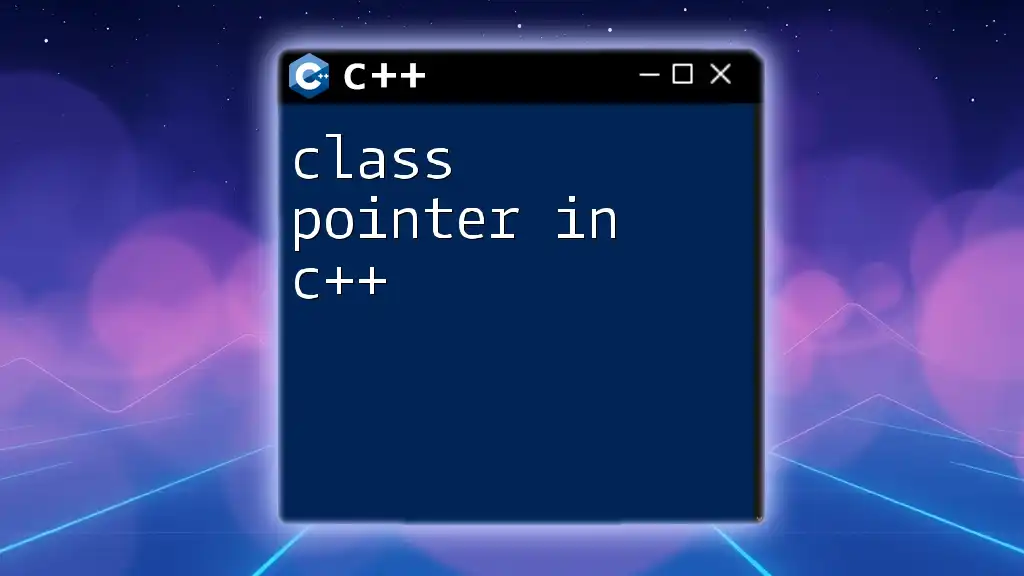
Practical Applications
Using Log Base 2 in Algorithms
Understanding the log base 2 is essential for analyzing algorithm efficiency, particularly in data structures like binary trees, where the height of the tree is logarithmic with respect to the number of elements.
Example: Binary Search
Binary search is a classic algorithm that demonstrates the usefulness of log base 2. The time complexity of binary search is \( O(\log_2(n)) \), meaning as the size of the dataset doubles, the number of comparisons only increases linearly, making it a highly efficient search method.
Here's a basic binary search implementation:
#include <iostream>
#include <vector>
#include <algorithm>
int binarySearch(const std::vector<int>& sortedArray, int target) {
int left = 0;
int right = sortedArray.size() - 1;
while (left <= right) {
int mid = left + (right - left) / 2;
if (sortedArray[mid] == target) {
return mid; // Target found
} else if (sortedArray[mid] < target) {
left = mid + 1;
} else {
right = mid - 1;
}
}
return -1; // Target not found
}
Performance Considerations
When considering performance, using the `log2` function is generally efficient; however, if computations are required for very large datasets, the custom implementation using bit manipulation can lead to performance gains by avoiding function call overhead.
Understanding these intricacies and knowing when to apply either method can greatly enhance your programming skills and algorithm implementations in C++.
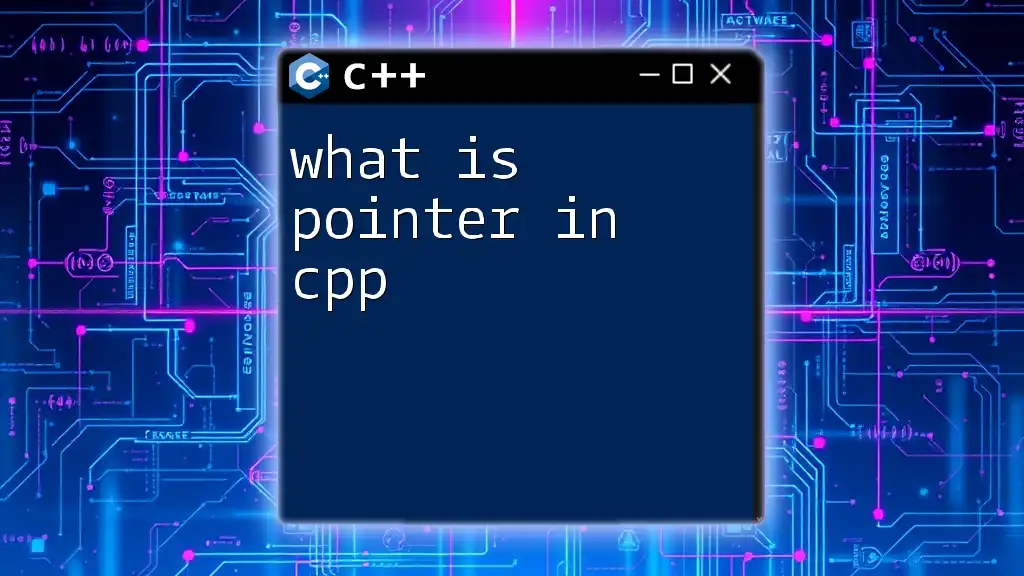
Conclusion
Calculating the log base 2 of an integer in C++ can be tackled directly using the `log2` function from the `<cmath>` library or by implementing your own function, enriching your understanding of logarithms and bit manipulation techniques. Mastering this concept not only aids in algorithm analysis but also strengthens your programming capabilities in scenarios where logarithmic calculations are prevalent.
As you practice, consider experimenting with various inputs and exploring other mathematical functions in C++. Engaging with these topics will deepen your knowledge and proficiency in C++ programming.