The error message "m_pinterceptor sap assert failure tenantinterceptor.cpp:210" indicates that there is an assertion failure related to a pointer in the SAP tenant interceptor code, usually occurring due to an unexpected null reference or invalid state in the tenant management logic.
Here’s a code snippet that might help you check for null references before using a pointer:
if (m_pinterceptor == nullptr) {
// Handle the error: m_pinterceptor is null
std::cerr << "Error: m_pinterceptor is null!" << std::endl;
// Additional error handling code here
} else {
// Proceed with normal operations on m_pinterceptor
}
Understanding Assert Failures
What is an Assert Failure?
An assert failure is a specific type of error that occurs in C++ programming when an assertion statement evaluates to false. Assertions serve as sanity checks within programs, allowing developers to catch bugs and logical errors during development. When an assert fails, it indicates that an assumption made by the programmer is not valid, which often leads to program crashes or unintended behavior.
Common Causes of Assert Failures
Understanding the causes behind assert failures is vital for effective debugging. Here are some prevalent reasons:
-
Logic Errors: These are mistakes in the flow of logic in the code. For example, if an assertion checks that a variable should never be `null`, but due to a flaw in the logic, it becomes `null`, this will trigger an assert failure.
-
Data Type Mismatches: C++ is a statically typed language. Type mismatches can cause an assert statement to fail unexpectedly. For instance, passing a float where an integer is expected can result in such failures.
-
Resource Mismanagement: Issues like memory leaks and problems with pointers, such as dereferencing null or dangling pointers, often lead to assert failures.
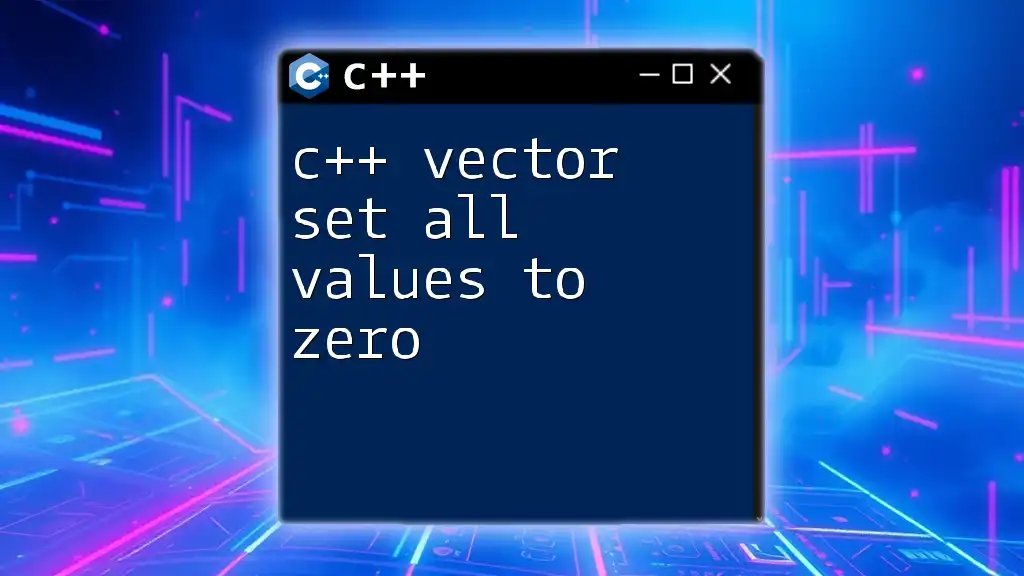
A Deep Dive into `m_pinterceptor sap assert failure tenantinterceptor.cpp:210`
What is `m_pinterceptor`?
Within the context of SAP applications, `m_pinterceptor` refers to a pointer or an object that acts as an interceptor for certain processes. Interceptors are essential for modifying or extending application behavior without altering the primary source code. They can intercept calls and manage data effectively.
The Significance of `tenantinterceptor.cpp`
The `tenantinterceptor.cpp` file plays a crucial role in managing the behavior of multi-tenant applications in SAP environments. This file typically contains the logic that allows different tenants (or users) to interact with shared resources while maintaining security and data integrity.
Analyzing the Error Message: `tenantinterceptor.cpp:210`
In the error message `tenantinterceptor.cpp:210`, the number 210 pinpoints the specific line in the code where the assertion failed. Understanding this line is crucial, as it often encapsulates the critical logic that could be causing the problem.
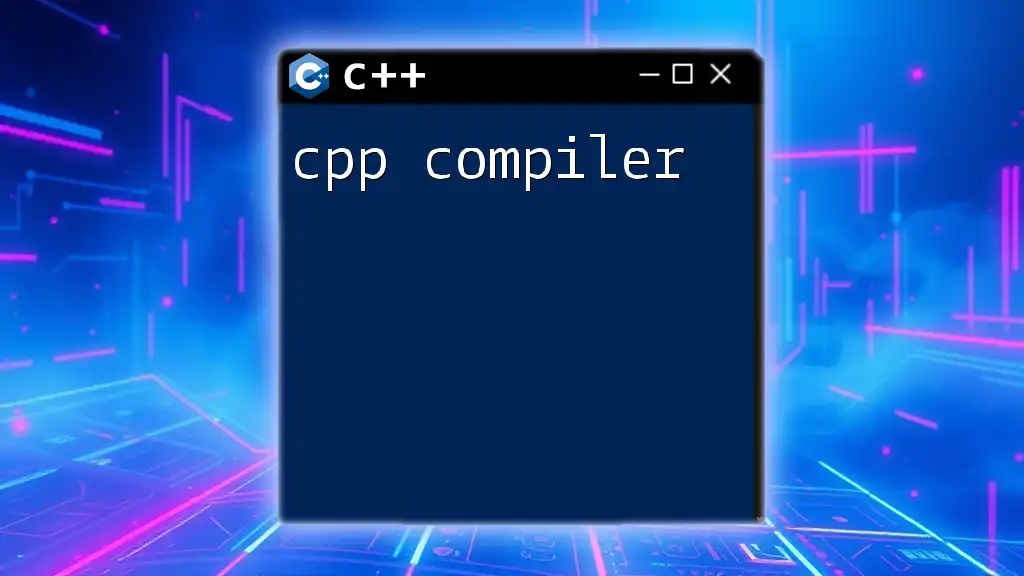
Troubleshooting the Assert Failure
Steps to Diagnose the Issue
The first step in troubleshooting an assert failure at `tenantinterceptor.cpp:210` is to thoroughly review the code on that line. Common patterns to investigate include:
- Check for any assumptions that the code makes about the state of objects or data types.
- Ensure that all pointers are properly initialized and used.
Example Code Snippet
Consider the following hypothetical snippet from `tenantinterceptor.cpp` that could be leading to the assert failure:
if (m_pinterceptor == nullptr) {
assert(m_pinterceptor != nullptr && "Interceptor pointer should not be null");
}
In this example, if `m_pinterceptor` is indeed `nullptr`, the assert will fire, resulting in an error message indicating where the issue occurs.
Common Fixes and Resolutions
To address common causes of assert failures:
-
Initialization: Ensure pointers like `m_pinterceptor` have been initialized before use. You can do this by checking the conditions under which they are assigned values.
-
Review Logical Conditions: Reassess the conditions leading to the assert statement. Logic that assumes a variable to have a specific state must be verified to avoid mismatches.
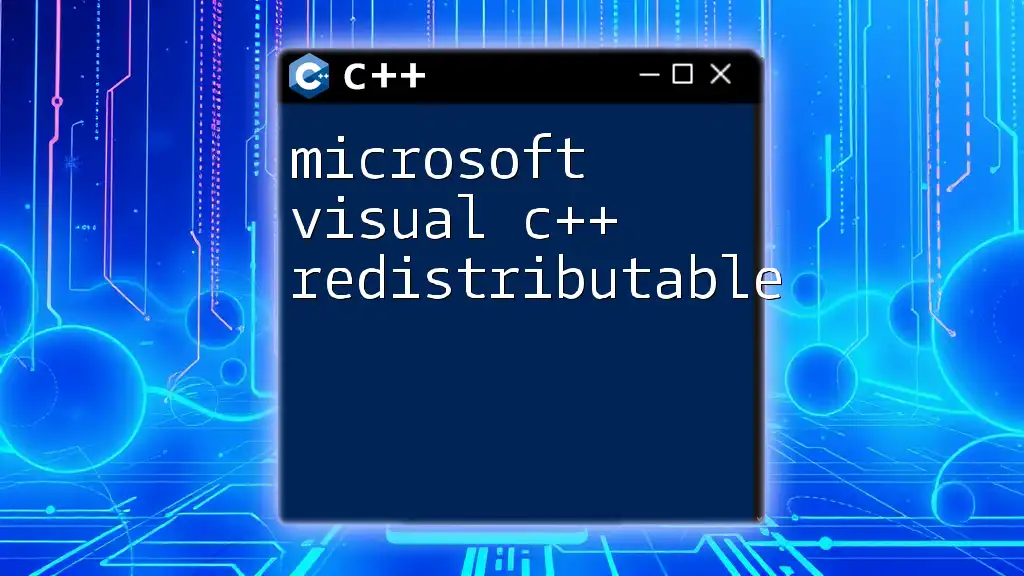
Best Practices in C++ Development
Implementing Safety Nets
Incorporating additional error handling measures can mitigate assert failures:
-
Exceptions: Use exceptions for error handling instead of relying solely on assert statements. This approach allows for more graceful recovery from errors.
-
Logging: Implement robust logging mechanisms to track the application state leading up to failures. This provides context during troubleshooting and helps avoid [similar scenarios](https://www.cppreference.com/).
Code Quality Assurance
Maintaining code integrity through regular audits and tests can significantly reduce the incidence of assert failures:
-
Conduct Code Reviews: Peer reviews help catch errors before the code gets into production, ensuring a fresh set of eyes checks logic and data flow.
-
Automated Testing: Implement unit tests to cover edge cases, allowing your asserts to be validated through rigorous testing.
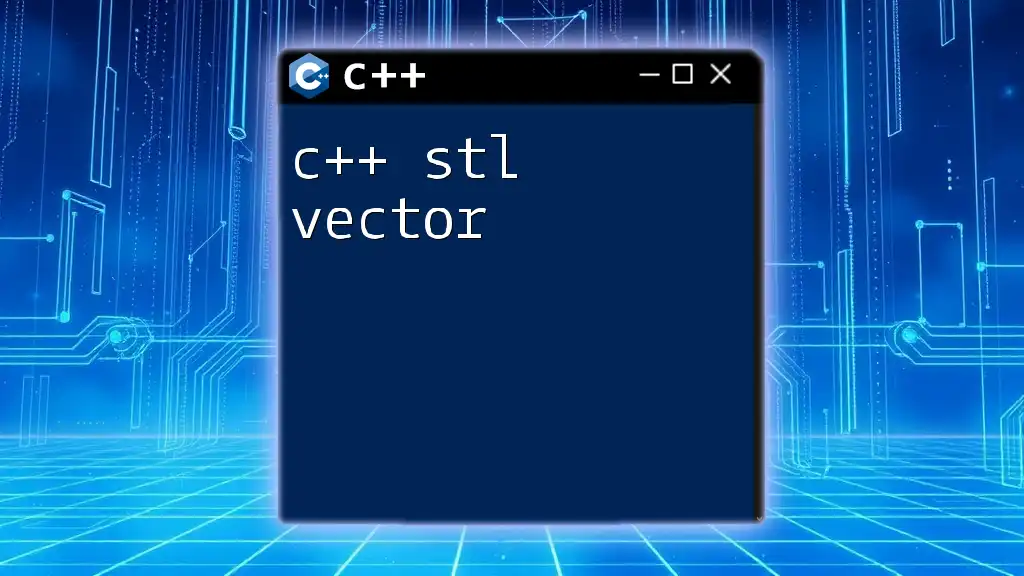
Conclusion
Understanding the mechanics behind `m_pinterceptor sap assert failure tenantinterceptor.cpp:210` is vital for any developer working within the SAP environment. Assert failures provide critical feedback about code assumptions and logic. By digging deep into the nature of these assertions and employing best practices, developers can enhance their code quality, reduce bugs, and facilitate better user experiences.
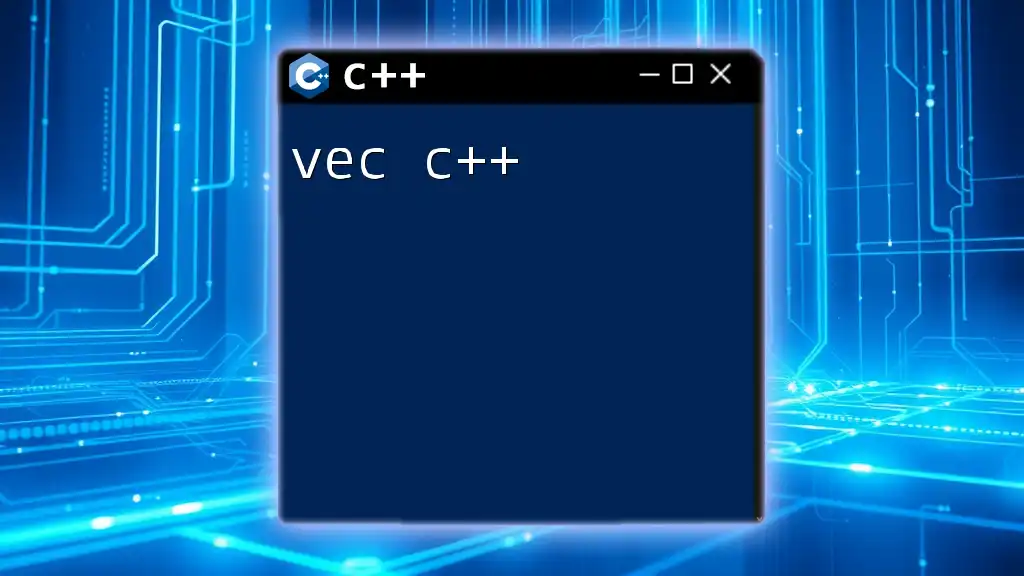
Additional Resources
For further exploration of this topic, consider diving into relevant C++ programming resources, books, and community platforms focused on SAP development. Engaging with the developer community can provide insights into common pitfalls and innovative solutions, making you better equipped to handle assert errors and optimize your C++ applications.