Templates in C++ should be used when you want to create functions or classes that can operate with any data type without the need for code duplication.
template <typename T>
T add(T a, T b) {
return a + b;
}
Understanding C++ Templates
What Are Templates?
Templates in C++ are a powerful feature that allows developers to write generic and reusable code. They enable you to define functions or classes with placeholders for types, which are specified when the template is instantiated. There are two primary types of templates:
- Class Templates: These allow you to create classes that can operate with any data type.
- Function Templates: These enable you to create functions that can perform actions on any data type.
For example, consider a simple function template for adding two numbers:
template<typename T>
T add(T a, T b) {
return a + b;
}
In this example, `T` serves as a placeholder for any data type, allowing the `add` function to be used with integers, floating-point numbers, or even custom types, thus showcasing the flexibility and reusability of templates.
The Benefits of Using Templates
Using templates in C++ provides several compelling advantages:
-
Code Reusability: One of the primary benefits of templates is that they allow you to write code that is usable in many different contexts without duplication. This means you can create functions or classes that work with any data type while maintaining a clean and efficient codebase.
-
Type Safety: When using templates, the data types are checked at compile time. This ensures that type mismatches are caught early, reducing runtime errors and improving code reliability.
-
Performance: In many cases, templates allow the compiler to optimize the generated code for specific types, leading to improved performance. Because templates are instantiated at compile time, there is less overhead compared to traditional polymorphism.
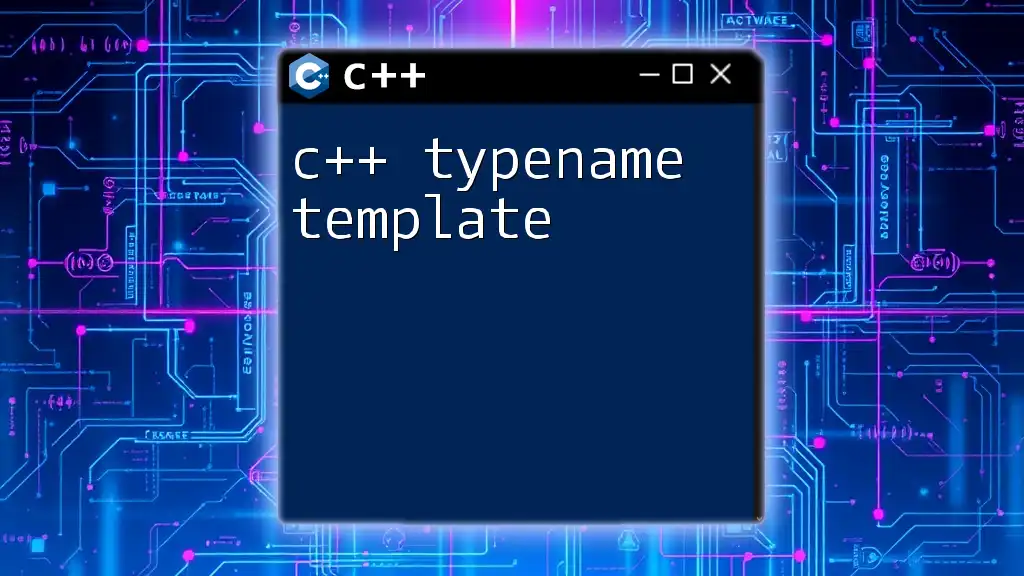
When to Use Templates
Generic Programming
Templates are integral to the concept of generic programming, where you define algorithms that work independently of specific data types. This approach enhances flexibility and reduces redundancy in code.
For instance, a template for a generic sorting function can be easily defined:
template<typename T>
void sort(T arr[], int size) {
// Sorting logic implemented here
}
In this example, the `sort` function can now sort arrays of different types, such as `int`, `float`, or even objects of user-defined classes, demonstrating how templates enable efficient code reuse.
When Flexibility Is Required
In scenarios where the types of inputs may vary significantly, templates shine. They allow functions and classes to accept parameters of different types without the need for overloading or rewriting code. This is particularly useful when implementing container classes or data structures.
For example, consider the `std::vector` container, which utilizes templates to allow storage of any data type:
std::vector<int> intVec;
std::vector<std::string> strVec;
Both lines declare vectors that can store integers and strings respectively, highlighting the adaptability provided by templates in C++.
Optimizing Performance in Libraries
Templates are critical for optimizing performance in library implementations. The Standard Template Library (STL) is an exemplary illustration of this principle. Containers and algorithms in STL, such as `std::pair`, utilize templates to provide maximum efficiency and flexibility:
template <class T1, class T2>
class Pair {
public:
T1 first;
T2 second;
};
Using templates in such a way allows `Pair` to hold any combination of types without sacrificing performance or requiring additional code.
Avoiding Code Duplication
Templates are particularly valuable when it comes to avoiding code duplication. Without templates, the same function may need to be defined multiple times for different types. For instance, consider two overloaded functions for multiplication:
int multiply(int a, int b);
double multiply(double a, double b);
Using a function template, the same logic can be distilled into a single definition:
template<typename T>
T multiply(T a, T b) {
return a * b;
}
This template eliminates redundancy and makes maintaining the code easier.
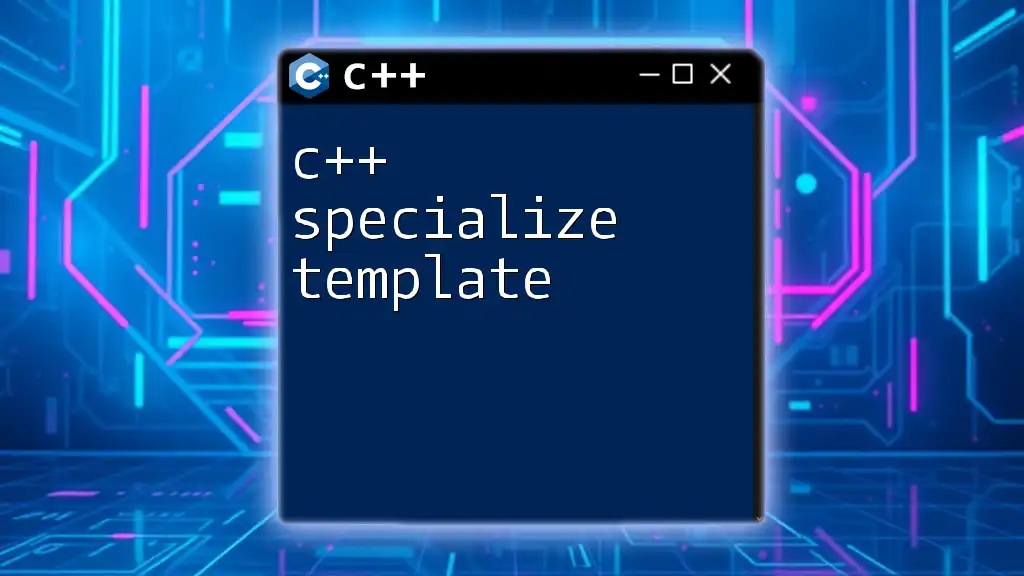
Common Examples of Template Usage
Template Classes
Creating a template class allows you to define data structures that can work with any type. Here’s a simple implementation of a Stack class template:
template <typename T>
class Stack {
private:
std::vector<T> elements; // Vector to hold stack elements
public:
void push(const T& element);
T pop();
};
This Stack class can be instantiated with any datatype, allowing for stacks of integers, strings, or even complex object types.
Template Specialization
Template specialization provides a way to customize the behavior of a template for specific types. This is useful when you want different implementations based on the type passed to the template.
For example, you can create a specialized version of a Stack for character pointers:
template <>
class Stack<char*> {
// Specialized implementation for char* here
};
This allows for distinct behavior or optimizations specific to handling character arrays or strings.
Template Metaprogramming
Template metaprogramming leverages templates to perform computations at compile-time, generating efficient and highly optimized code. A classic example involves calculating factorials:
template<int N>
struct Factorial {
static const int value = N * Factorial<N - 1>::value;
};
template<>
struct Factorial<0> {
static const int value = 1;
};
This showcases how templates can be used to create compile-time constant expressions, which can significantly speed up function execution and reduce overhead.
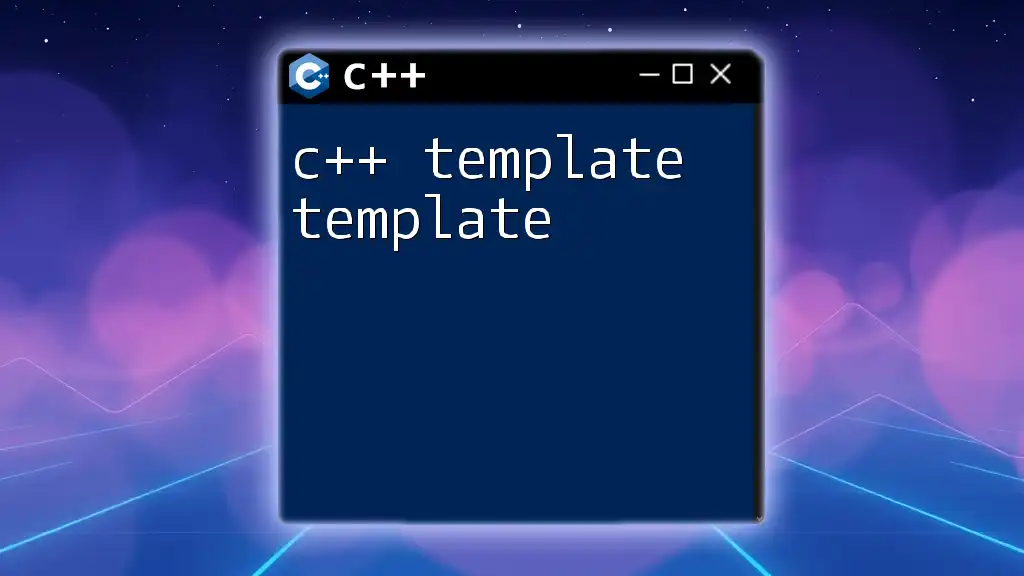
Limitations and Drawbacks of Templates
Increased Compilation Times
While templates provide numerous benefits, they can also lead to longer compilation times. This occurs because the compiler generates code for every unique instantiation of a template type. To mitigate this issue, consider limiting the number of template parameters or using implementation files (`.cpp`) for template definitions to separate the code and speed up compilation.
Debugging Complexities
Debugging code that relies heavily on templates can be complex due to often cryptic compiler-generated error messages. Such scenarios require a deep understanding of both templates and the specific types involved. To assist with debugging, it helps to provide explicit type arguments during instantiation or to utilize simpler constructs that do not overly complicate template usage.
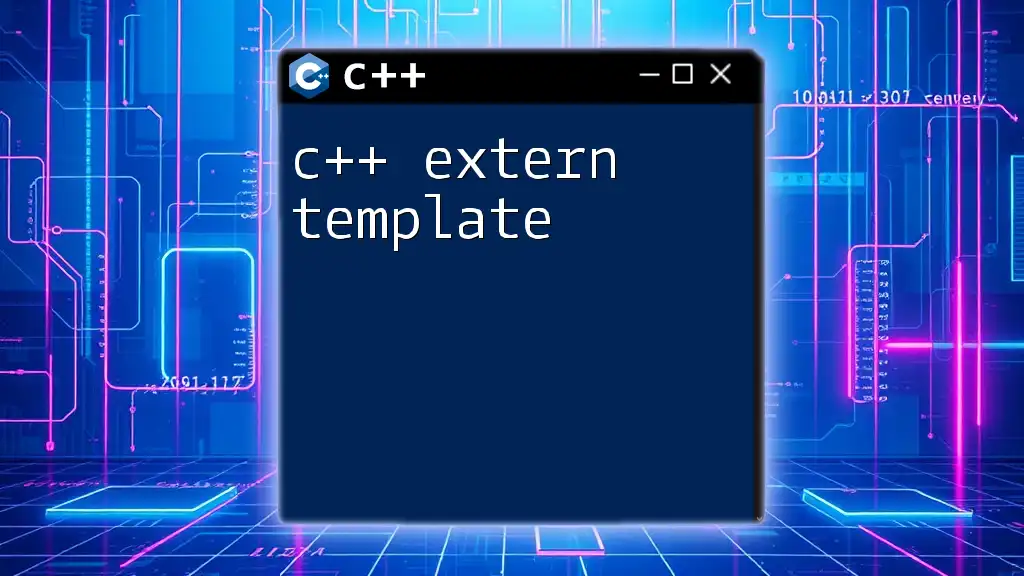
Conclusion
In summary, understanding C++ when to use templates is crucial for any aspiring C++ developer. Templates provide enhanced flexibility, type safety, and significant opportunities for code reuse. However, they also come with their own set of challenges, which require careful consideration. By recognizing situations where templates are most effective, developers can make informed decisions that lead to cleaner and more efficient C++ code. Use templates wisely in your next project to unlock their full potential!