The error "exception in invoking authentication handler unidentifiable C++ exception" typically indicates that an unexpected exception occurred during the authentication process, which can result from improper error handling or issues in the authentication code.
Here's a code snippet demonstrating basic error handling for a C++ authentication process:
#include <iostream>
#include <stdexcept>
void authenticateUser(bool isAuthenticated) {
if (!isAuthenticated) {
throw std::runtime_error("Authentication failed");
}
std::cout << "User authenticated successfully!" << std::endl;
}
int main() {
try {
authenticateUser(false); // Simulating failed authentication
} catch (const std::exception &e) {
std::cerr << "Exception caught: " << e.what() << std::endl;
}
return 0;
}
Understanding C++ Exceptions
What is an Exception in C++?
An exception in C++ is an event that disrupts the normal flow of a program when an error occurs. Exception handling allows developers to manage unexpected situations gracefully. By employing mechanisms like `try`, `catch`, and `throw`, developers can encapsulate error-prone code and respond appropriately to exceptions. This is particularly essential in creating robust applications, as it allows for cleaner error management and improved program stability.
Types of Exceptions
C++ provides built-in exceptions as part of its standard library, allowing developers to handle various types of errors efficiently:
- Standard Exceptions: These include `std::exception`, `std::runtime_error`, and many more, providing standardized ways to manage errors.
- User-defined Exceptions: Developers can create their own exception classes to handle specific application errors that may not fit into the standard exceptions. This custom approach enables more granular control over error behavior and messaging.
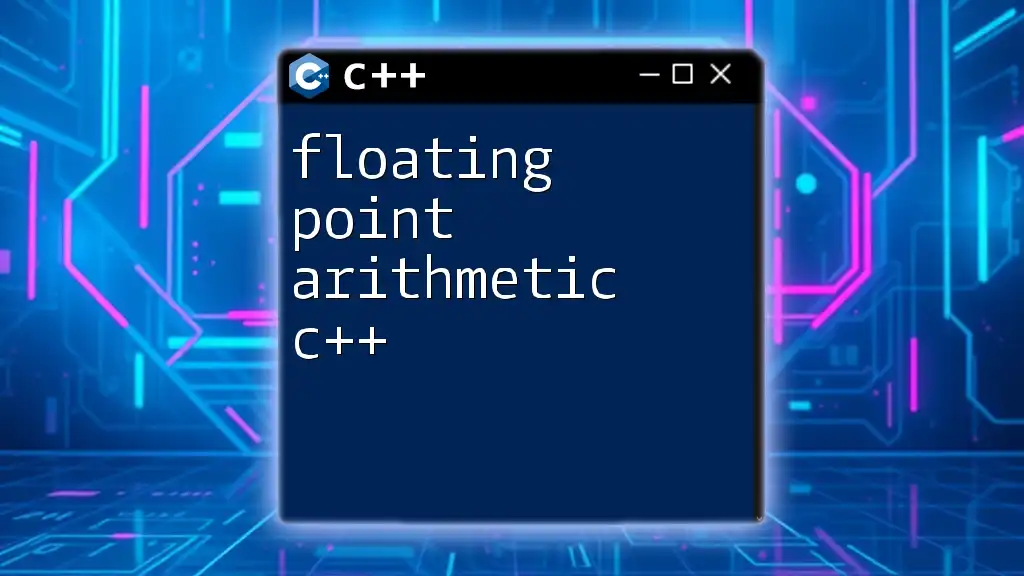
The Role of Authentication Handlers
What is an Authentication Handler?
An authentication handler is a crucial component within applications responsible for verifying user identities. It serves as a gatekeeper, ensuring that only authorized users gain access to sensitive resources. By integrating seamlessly with authentication protocols, these handlers are the backbone of secure applications.
Common Tasks of Authentication Handlers
Authentication handlers typically perform numerous tasks, including:
- User validation: Confirming whether credentials provided by users match those stored within the database.
- Session management: Handling user sessions through tokens or sessions to maintain a continuous login state.
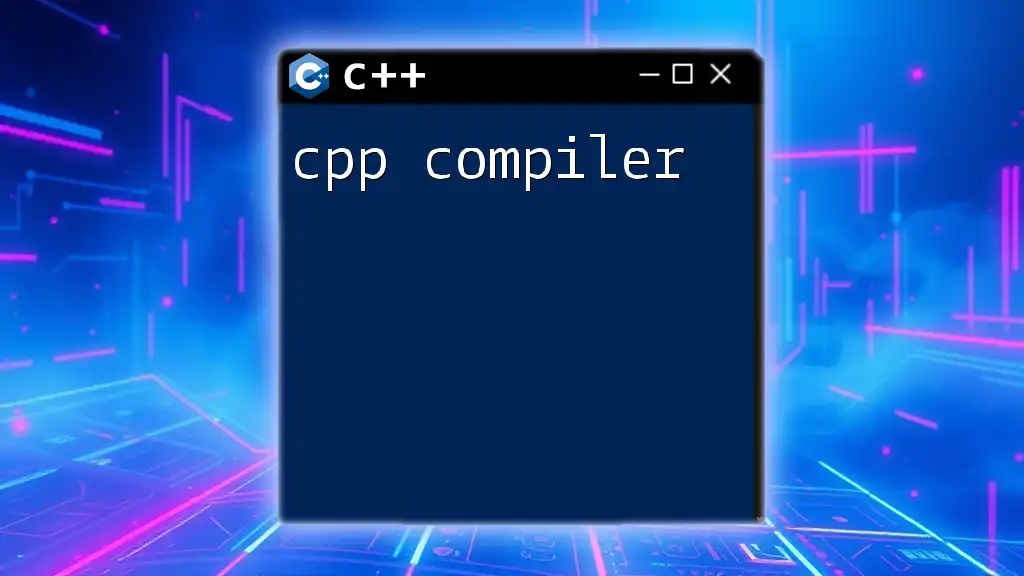
The Issue of "Unidentifiable C++ Exceptions"
What Does "Unidentifiable Exception" Mean?
An unidentifiable C++ exception refers to an error that arises during execution but cannot be classified into known exceptions. This ambiguity often makes debugging extremely challenging, as developers cannot pinpoint the exact cause of the error.
Impact on Authentication Handlers
The occurrence of unidentifiable exceptions can have serious repercussions on the authentication process:
- Performance Issues: These exceptions can lead to increased latency as the system attempts to process authentication tasks, resulting in a sluggish response for users.
- User Experience: When users encounter generic error messages without specific guidance, it can lead to confusion and frustration, negatively impacting their overall experience.
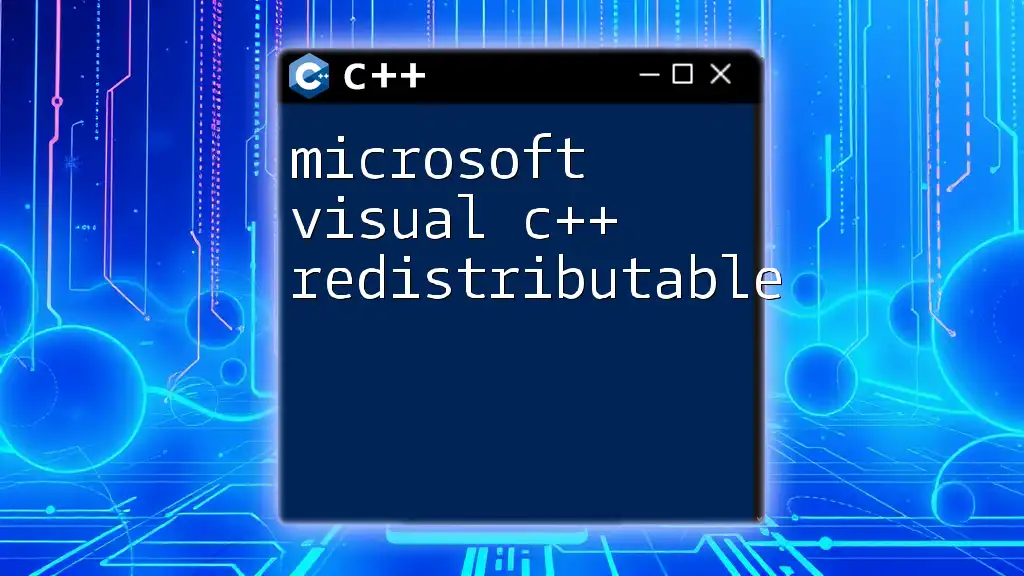
Diagnosing the "Unidentifiable C++ Exception"
Debugging Techniques
To tackle the problem, leveraging debugging tools is essential. Tools such as gdb (GNU Debugger) or the built-in debugger in IDEs like Visual Studio can help trace exceptions back to their origins, allowing developers to address the root cause effectively.
Example Code Snippet: Basic Exception Handling
try {
// code that might throw exceptions
} catch (const std::exception& e) {
std::cerr << "An exception occurred: " << e.what() << '\n';
}
This structure showcases a fundamental approach to catching standard exceptions, demonstrating that while the flow can be interrupted due to issues, graceful handling is possible.
Importance of Stack Traces
Obtaining and interpreting stack traces can be instrumental in debugging. Stack traces provide a snapshot of the call stack at the moment an exception occurred, allowing developers to trace back through the layers of function calls. Tools such as `backtrace` in Linux can facilitate this process, giving insights into where the error originated.
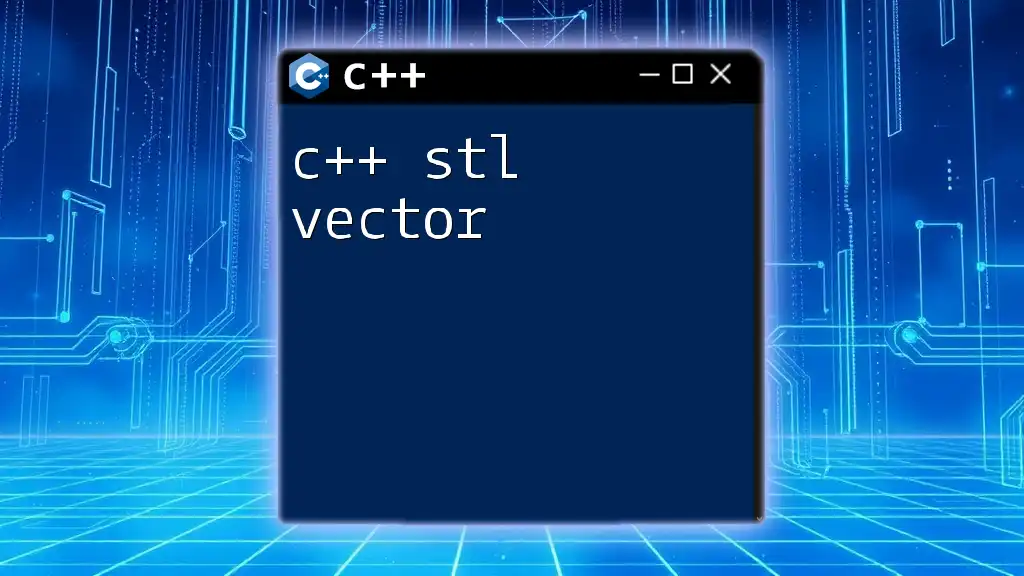
Handling Exceptions in Authentication Handlers
Best Practices for Error Handling
To effectively manage exceptions related to authentication, developers should adhere to best practices:
- Using `try-catch` blocks: Properly structuring your code with `try-catch` ensures that unexpected errors are caught, preventing them from crashing the application.
- Implementing fallback options helps maintain basic functionality even when errors are encountered.
Example Code Snippet: Catching Unidentifiable Exceptions
try {
authenticationHandler.authenticate(user);
} catch (const std::exception& e) {
// Log the exception for analysis
logError(e.what());
} catch (...) {
// Catch any unidentifiable exceptions
logError("An unidentifiable exception occurred while authenticating.");
}
In this example, the `catch (...)` block ensures that even unknown exceptions can be captured and logged for future analysis, thus fortifying the application's resilience.
Creating Custom Exceptions for Better Clarity
Creating user-defined exceptions is a powerful method to make exception handling more comprehendible. By providing specific error messages and handling, developers can drastically enhance the clarity of their authentication processes.
Example Code Snippet: Custom Exception Class
class AuthException : public std::exception {
public:
const char* what() const noexcept override {
return "Authentication failure due to an unknown reason.";
}
};
// Using the custom exception
throw AuthException();
This example illustrates how creating a custom exception allows developers to define specific scenarios, giving them control over the messaging and handling of authentication issues.
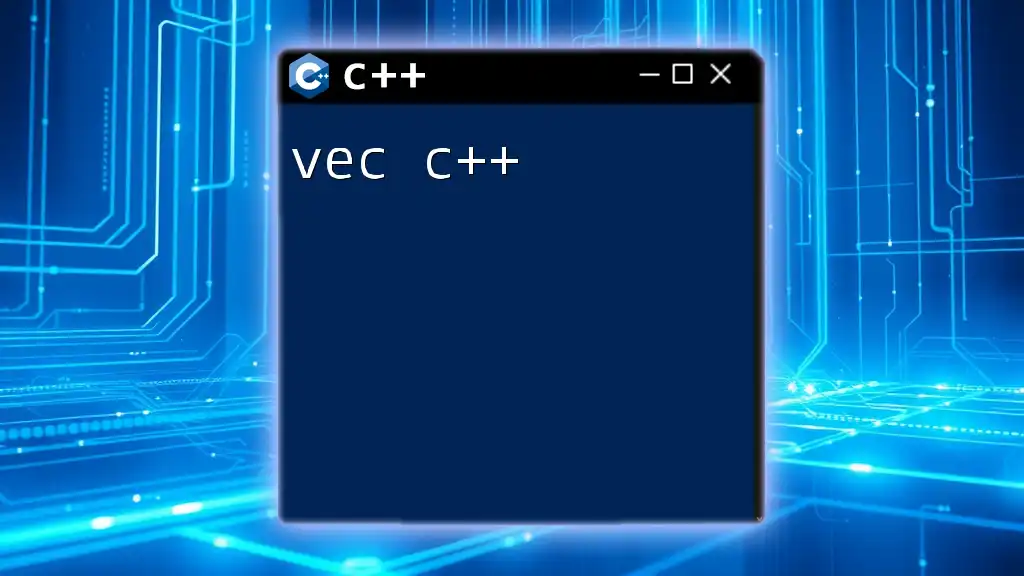
Testing and Validating Authentication Handlers
Writing Unit Tests for Exception Handling
Unit testing forms an essential part of quality assurance in software development. By writing tests that specifically check for exception handling, developers can ensure that their authentication handlers respond correctly under various conditions.
Example Code Snippet: Unit Test Example
TEST(AuthHandlerTest, AuthenticationThrowsException) {
EXPECT_THROW(authenticationHandler.authenticate(invalidUser), AuthException);
}
This unit test verifies that an AuthException is thrown when an invalid user attempts authentication, ensuring the robustness of the authentication handler.
Logging and Monitoring
Effective logging practices are crucial in identifying and understanding exceptions post-occurrence. Utilizing logging frameworks such as log4cpp allows developers to capture exception details, facilitating easier diagnosis during future troubleshooting.
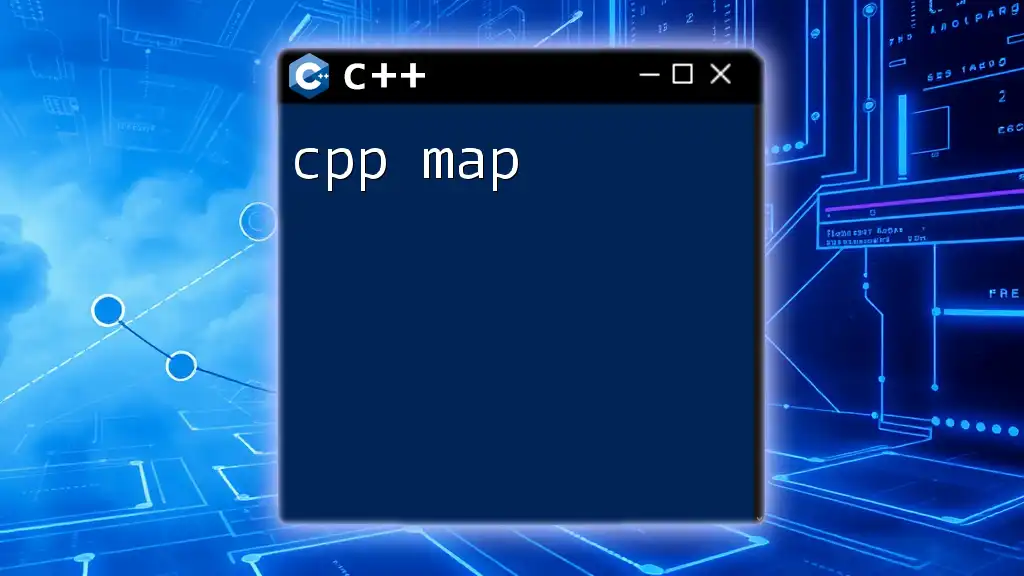
Conclusion
Recap of Key Points
In summary, encountering an "exception in invoking authentication handler unidentifiable C++ exception" can pose significant challenges. Understanding the nature of exceptions, leveraging effective debugging techniques, and implementing structured exception handling can enhance the reliability of application authentication processes. By creating custom exceptions and committing to robust logging, developers improve both the stability and user experience of their applications.
Additional Resources
To further deepen your knowledge on the subject, consider exploring additional reading materials and tools that specialize in C++ exception handling, debugging, and authentication best practices.